[ad_1]
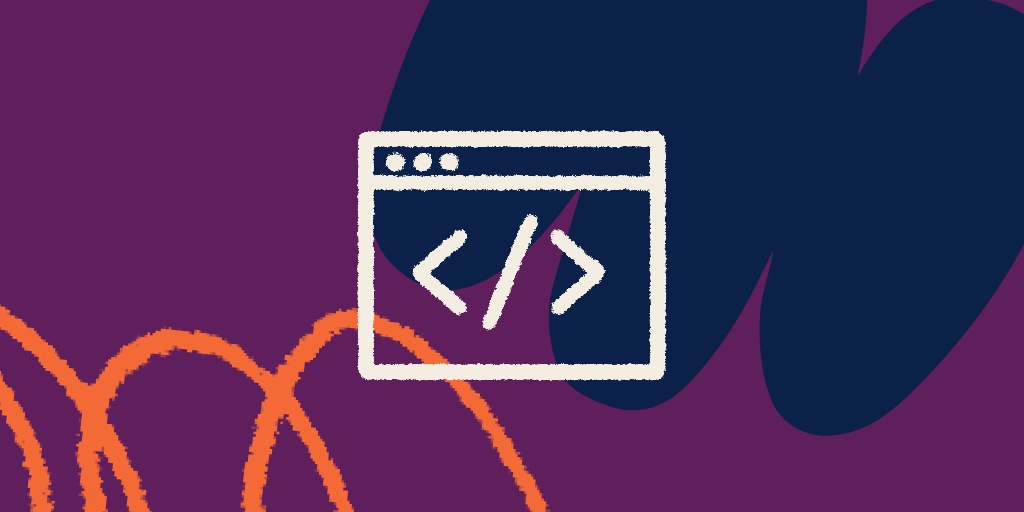
by Adam Berkan
Khan Academy is finishing a big task to go our backend from Python to Go. Although the most important objective of the challenge was to migrate off an obsolete system, we saw an prospect to make improvements to our code over and above just a straight port.
One big matter we required to improve was the implicit dependencies that were all about our Python codebase. Accessing the recent request or existing user was done by calling world-wide functions. Also, we related to other inner companies and exterior features, like the databases, storage, and caching layer, by international capabilities or international decorators.
Applying globals like this manufactured it challenging to know if a block of code touched a piece of information or named out to a company. It also difficult code tests since all the implicit dependencies wanted to be mocked out.
We deemed a amount of achievable options, which include passing in anything as parameters or utilizing the context
to maintain all dependencies, but each and every solution had failings.
In this article, I’m going to describe how and why we solved these difficulties by developing a statically typed context. We extended the context object with functions to access these shared methods, and features declare interfaces that exhibit which features they involve. The result is we have dependencies explicitly stated and confirmed at compile time, but it’s continue to quick to simply call and take a look at a purpose.
func DoTheThing(
ctx interface
context.Context
RequestContext
DatabaseContext
HttpClientContext
SecretsContext
LoggerContext
,
detail string,
) ...
I’ll wander through the various concepts we considered and exhibit why we settled on this answer. All of the code illustrations from this post are out there at https://github.com/Khan/typed-context. You can discover that repository to see working examples and the specifics of how statically typed contexts are carried out.
Attempt 1: Globals
Let’s start out with a motivating example:
func DoTheThing(issue string) error
// Locate User Essential from request
userKey, err := request.GetUserKey()
if err != nil return err
// Lookup Person in database
user, err := databases.Read(userKey)
if err != nil return err
// It's possible write-up an http if can do the matter
if user.CanDoThing(thing)
err = httpClient.Write-up("www.dothething.case in point", consumer.GetName())
return err
This code is fairly clear-cut, handles errors, and even has responses, but there are a couple significant troubles. What is ask for
right here? A world-wide variable!? And where by do database
and httpClient
arrive from? And what about any dependencies that people functions have?
Below are some good reasons why we really do not like world-wide variables:
- It is difficult to trace in which dependencies are employed.
- It is tough to mock out dependencies for testing given that each and every take a look at takes advantage of the exact globals.
- We just cannot operate concurrently versus different information.
Hiding all these dependencies in globals would make the code difficult to follow. In Go, we like to be express! Instead of implicitly relying on all these globals, let’s test passing them in as parameters.
Endeavor 2: Parameters
func DoTheThing(
factor string,
ask for *Ask for,
databases *Database,
httpClient *HttpClient,
techniques *Insider secrets,
logger *Logger,
timeout *Timeout,
) error
// Locate Consumer Key from request
userKey, err := request.GetUserKey()
if err != nil return err
// Lookup User in database
user, err := databases.Examine(userKey, techniques, logger, timeout)
if err != nil return err
// Possibly put up an http if can do the thing
if person.CanDoThing(issue)
token, err := ask for.GetToken()
if err != nil return err
err = httpClient.Write-up("www.dothething.instance", consumer.GetName(), token, logger)
return err
return nil
All of the functionality that is demanded to DoTheThing
is now extremely evident, and it’s apparent which ask for
is staying processed, which databases
is remaining accessed, and which strategies
the database is employing. If we want to exam this operate, it’s effortless to see how to pass in mock objects.
Sad to say the code is now extremely verbose. Some parameters are prevalent to almost every purpose and will need to be passed everywhere: ask for
, logger
, and tricks
, for case in point. DoTheThing
has a bunch of parameters that are only there so that we can go them on to other functions. Some features may well will need to take dozens of parameters to encompass all the functionality they want.
When every perform normally takes dozens of parameters, it’s hard to get the parameter buy correct. When we want to move in mocks, we want to create a big range of mocks and make absolutely sure they are suitable with each individual other.
We should really probably be examining every single parameter to make sure it is not nil, but in observe a lot of builders would just hazard panicking if the caller improperly passes nils.
When we include a new parameter to a purpose, we have to update all the phone web sites, but the contacting features also need to have to check if they previously have that parameter. If not, they need to have to incorporate it as a parameter of their very own. This final results in big amounts of non-automatable code churn.
1 opportunity twist on this plan is to build a server
item that bundles a bunch of these dependencies together. This method can reduce the selection of parameters, but now it hides just which dependencies a purpose basically desires. There’s a tradeoff among a massive number of compact objects and a number of massive ones that bundle together a bunch of dependencies that perhaps aren’t all employed. These objects can become all-potent utility classes, which negates the value of explicitly listing dependencies. The entire object will have to be mocked even if we only count on a smaller piece of it.
For some of this performance, like timeouts and the request, there is a normal Go solution. The context
library supplies an object that holds details about the recent ask for and supplies functionality all over handling timeouts and cancellation.
It can be even more extended to keep any other item that the developer wishes to move all around in all places. In apply, a great deal of code bases use the context as a catch-all bin that retains all the frequent objects. Does this make the code nicer?
Try 3: Context
func DoTheThing(
ctx context.Context,
thing string,
) error
// Discover Person Vital from ask for
userKey, err := ctx.Value("request").(*Request).GetUserKey()
if err != nil return err
// Lookup Person in databases
person, err := ctx.Worth("database").(*Databases).Go through(ctx, userKey)
if err != nil return err
// Perhaps publish an http if can do the factor
if person.CanDoThing(detail)
err = ctx.Price("httpClient").(*HttpClient).
Put up(ctx, "www.dothething.example", person.GetName())
return err
return nil
This is way smaller than listing every little thing, but the code is incredibly prone to runtime panics if any of the ctx.Price(...)
calls returns a nil or a benefit of the wrong sort. It is difficult to know which fields need to have to be populated on ctx
prior to this is identified as and what the anticipated style is. We really should most likely test these parameters.
Attempt 4: Context, but safely
func DoTheThing(
ctx context.Context,
detail string,
) mistake
So now we’re thoroughly examining that the context has all the things we have to have and dealing with glitches appropriately. The one ctx
parameter carries all the frequently employed operation. This context can be developed in a smaller selection of centralized spots for various scenarios (e.g., GetProdContext()
, GetTestContext()
).
Sad to say, the code is now even more time than if we passed in every thing as a parameter. Most of the extra code is tedious boilerplate that tends to make it tougher to see what the code is really carrying out.
This alternative does permit us get the job done on concurrent requests independently (every with its individual context), but it still suffers from a good deal of the other challenges from the globals alternative. In specific, there is no quick way to explain to what performance a perform demands. For instance, it’s not obvious that ctx
desires to consist of a “secret
” when you call datastore.Get
and that as a result it’s also vital when you get in touch with DoTheThing
.
This code suffers from runtime failures if the context is lacking required performance. This can lead to glitches in creation. For illustration, if we CanDoTheThing
almost never returns correct, we might not recognize this operate requirements httpClient
right until it begins failing. There’s no simple way at compile time to ensure that the context will usually incorporate everything it desires.
Our Alternative: Statically Typed Context
What we want is anything that explicitly lists our function’s dependencies but doesn’t have to have us to list them at each get in touch with site. We want to validate all dependencies at compile time, but we also want to be in a position to increase a new dependency without the need of a enormous handbook code change.
The option we have intended at Khan Academy is to lengthen the context item with interfaces representing the shared functionality. Each individual operate declares an interface that describes all the functionality it demands from the statically typed context. The purpose can use the declared features by accessing it through the context.
The context is treated usually just after the operate signature, getting passed alongside to other capabilities. But now the compiler assures that the context implements the interfaces for each and every operate we get in touch with.
func DoTheThing(
ctx interface
context.Context
RequestContext
DatabaseContext
HttpClientContext
SecretsContext
LoggerContext
,
thing string,
) error
// Come across Person Crucial from ask for
userKey, err := ctx.Request().GetUserKey()
if err != nil return err
// Lookup User in databases
consumer, err := ctx.Databases().Read(ctx, userKey)
if err != nil return err
// Possibly post an http if can do the factor
if user.CanDoThing(matter)
err = ctx.HttpClient().Article(ctx, "www.dothething.instance", consumer.GetName())
return err
The overall body of this perform is practically as simple as the authentic function using globals. The purpose signature lists all the required performance for this code block and the capabilities it phone calls. Detect that contacting a operate these types of as ctx.Datastore().Browse(ctx, …)
doesn’t involve us to improve our ctx
, even though Go through
only necessitates a subset of the performance.
When we need to call a new interface that was not formerly component of our statically typed context, we will need to include the interface with a one line to our perform signature. This documents the new dependency and enables us to phone the new perform on the context.
If we experienced callers who really do not have the new interface in their context, they’ll get an mistake message describing what interface they are lacking, and they can add the identical context to their signature. The developer has a likelihood when earning the improve to make absolutely sure the new dependency is suitable. A modify like this can occasionally ripple up the stack, but it’s just a a single line modify in just about every impacted purpose right until we achieve a stage that even now has that interface. This can be a little bit bothersome for deep get in touch with stacks, but it is also some thing that could be automated for significant alterations.
The interfaces are declared by just about every library and normally consist of a solitary phone that returns either a piece of data or a customer item for that functionality. For illustration, here’s the ask for
and databases
context interfaces in the sample code.
variety RequestContext interface
Request() *Ask for
context.Context
kind DatabaseInterface interface
Read through(
ctx interface
context.Context
SecretsContext
LoggerContext
,
key DatabaseKey,
) (*Consumer, mistake)
variety DatabaseContext interface
Databases() DatabaseInterface
context.Context
We have a library that supplies contexts for diverse scenarios. In some situations, these types of as at the start of our request handlers, we have a essential context.Context
and want to upgrade it into a statically typed context.
func GetProdContext() ProdContext ...
func GetTestContext() TestContext ...
func Update(ctx *context.Context) ProdContext ...
These prebuilt contexts typically meet up with all the Context Interfaces in our code base and can thus be passed to any perform. The ProdContext
connects to all our products and services in manufacturing, even though our TestContext
makes use of a bunch of mocks that are designed to function appropriately alongside one another.
We also have distinctive contexts that are for our developer surroundings and for use inside of cron work. Each context is implemented in another way, but all can be handed to any operate in our code.
We also have contexts that only implement a subset of the interfaces, this sort of as a ReadOnlyContext
that only implements the read through-only interfaces. You can go it to any purpose that does not demand writes in its Context Interfaces. This ensures, at compile time, inadvertent writes are not possible.
We have a linter to guarantee that each individual perform declares the minimum interface needed. This assures that features don’t just declare they need “everything.” You can uncover a model of our linter in the sample code.
Summary
We’ve been working with statically typed contexts at Khan Academy for two decades now. We have around a dozen interfaces functions can rely on. They’ve made it very easy to keep track of how dependencies are applied in our code and are also helpful for injecting mocks for tests. We have compile time assurance that all capabilities will be readily available right before they are applied.
Statically typed contexts aren’t usually amazing. They are much more verbose than not declaring your dependencies, and they can involve fiddling with your context interface when you “just want to log one thing,” but they also help you save perform. When a purpose wants to use new performance it can be as simple as declaring it in your context interface and then working with it.
Statically typed contexts have eradicated whole classes of bugs. We by no means have uninitialized globals or missing context values. We never ever have something mutate a world and break later requests. We never have a perform that unexpectedly phone calls a service. Mocks often enjoy properly jointly for the reason that we have a corporation-extensive conference for injecting dependencies in take a look at code.
Go is a language that encourages being explicit and working with static forms to improve maintainability. Applying statically typed contexts lets us obtain people ambitions when accessing worldwide assets.
If you’re also fired up about this option, test out our professions site. As you can picture, we’re selecting engineers!
[ad_2]
Supply hyperlink